Web scraping sous Python (1/10) | Rudiments
Premier pas de web scraping
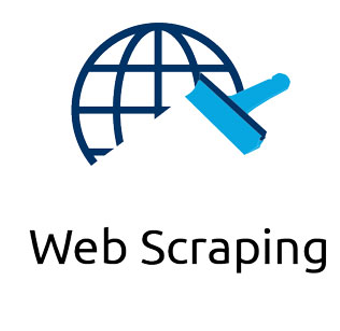
Urlopen
Approche basique en utilisant la classe urlopen
du module request
from urllib.request import urlopen
html = urlopen('http://pythonscraping.com/pages/page1.html')
print(html.read())
<html>
\n<head>
\n
<title>A Useful Page</title>
\n</head
>\n
<body>
\n
<h1>An Interesting Title</h1>
\n
<div>
\nLorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod
tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim
veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea
commodo consequat. Duis aute irure dolor in reprehenderit in voluptate
velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat
cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id
est laborum.\n
</div>
\n
</body>
\n
</html>
\n
BeautifulSoup
Par rapport à urlopen, on n’a pas besoin d’appeler read
pour accéder au contenu de page web.
Il est à noter que la deuxième option lors de la création d’un objet BeautifulSoup précise le parseur qui est 'html.parser'
dans notre cas.
A sa place, le parseur lxml
montre plus d’avantage que html.parser
dans le sens où il fonctionne mieux face aux HTML codes mal formés tels que des balises non fermées, mal imbriquées ou oublées. De plus, il est plus rapide que l’autre même si la vitesse n’est pas forcément un avantage dans web scraping car le plus important goulet serait souvent la vitesse du réseau elle-même.
from bs4 import BeautifulSoup
html = urlopen('http://www.pythonscraping.com/pages/page1.html')
bs = BeautifulSoup(html.read(), 'html.parser')
print(bs.h1)
<h1>An Interesting Title</h1>
Même output avec différentes expressions
html = urlopen('http://www.pythonscraping.com/pages/page1.html')
bs = BeautifulSoup(html, 'html.parser')
Expression 1
print(bs.h1)
Expression 2
print(bs.html.h1)
Expression 3
print(bs.html.body.h1)
Expression 4
print(bs.body.h1)
Gérer l’erreur d’adresse de page Web
L’erreur HTTP peut être “404 Page Not Found”, “500 Internal Server Error”, etc.
from urllib.error import HTTPError
from urllib.error import URLError
try:
html = urlopen("http://www.pythonscraping.com/pages/page1.html22")
except HTTPError as e: # this is actually a more layman understanding of URLError. HTTPError is a predefined name so that you can't replace it with other names
print(e)
except URLError as e:
print("The server could not be found!")
# the server error means there is no such website as This HTTP error may be “404 Page Not Found,” “500 Internal Server Error,” and so forth
else:
print(html.read())
HTTP Error 404: Not Found
Ecrire une fonction pour l’erreur url
def getTitle(url):
try:
html = urlopen(url)
except HTTPError as e:
return None
try:
bsObj = BeautifulSoup(html.read(), "lxml")
title = bsObj.body.h1
except AttributeError as e:
return None
return title
# check if an element is none
title = getTitle("http://www.pythonscraping.com/pages/page1.html")
if title == None:
print("Title could not be found")
else:
print(title)
<h1>An Interesting Title</h1>